Advanced Multi Stage Bot
In this tutorial, we will build a more complex voice bot/agent that is based on multiple stages.
Why using multi stage bots
In theory, it is possible to build complex call flows using a single stage just with prompting. However, LLMs often tend to hallizunate and may take different than the desired paths. In order to avoid that, we can use several stages, where each stage represents a separate dialog in a call session. Also, each stage comes with its own prompt where we can tell/instruct the LLM exactly what to do.
Let's image an apppointment voice bot. The voicebot first queries the user if he wants to book or cancel a booking for an appointment. In case the user wants to book an appointment, in the first stage, the bot would ask what type of appointment the user wants to book and at what day, while in the second stage, the bot would collect all information about the booking person such as name, email, phone number.
While in the cancellation case, the bot would first query the caller for his/her name, in order to indentify the user, and then retrieve existing bookings, listing them and querying the user what exact appointment he or she would like to cancel.
In order to illustrate the multi stage behavior, we will extend the single stage bot from the first tutorial but split up the information collection in two stages, i.e., first querying the caller for his/her name, and move then to the next stage for querying his/her date of birth.
Setting Up/Configuring The Bot
First, we define/use the Welcome stage and define the following prompt:
You are voice bot that collects some user/customer data.
You respond in German.
You respond in a short, very conversational friendly style.
Respond to any question which is outside of the purpose of collecting
the customer's name and birthday with I do not know.
Do not make up any answers.
Greet the user and ask the user for his name.
We then add a second stage and call this stage Stage DOB
but leave it blank for now.
Next, we define in the tools section a function collectName
with the following parameter: name
as string
and provide an appropriate description.
The tools should then be configured as follows:
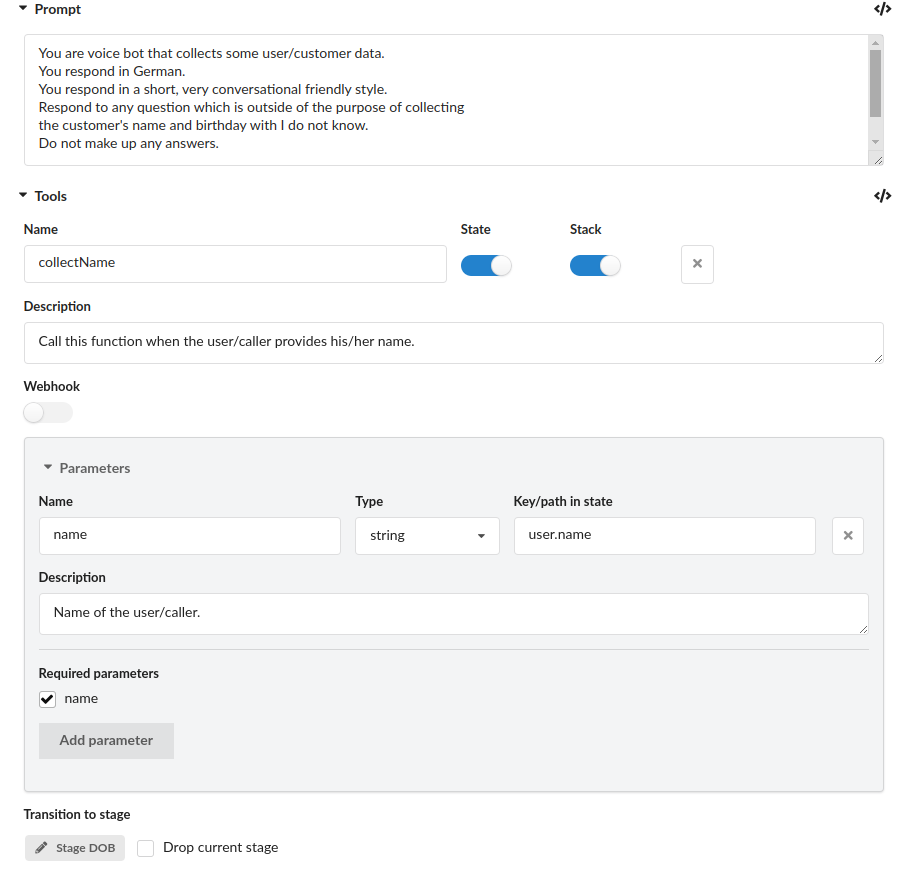
Note that we also define a key/path where the collected information, i.e., the name should be stored in the state. And, we also define a transition to the previously created Stage DOB
stage since we want to collect the date of birth afterwards.
Finally, we need to provide the implementation of the collectName
function that we defined in the tools section. In our example, we simply do not return anything since the voice bot will immediately ask for the date of birth as a next step.
function collectName(args) {
return { "text": "" };
}
First, we define/use the DOB stage and define the following tools:
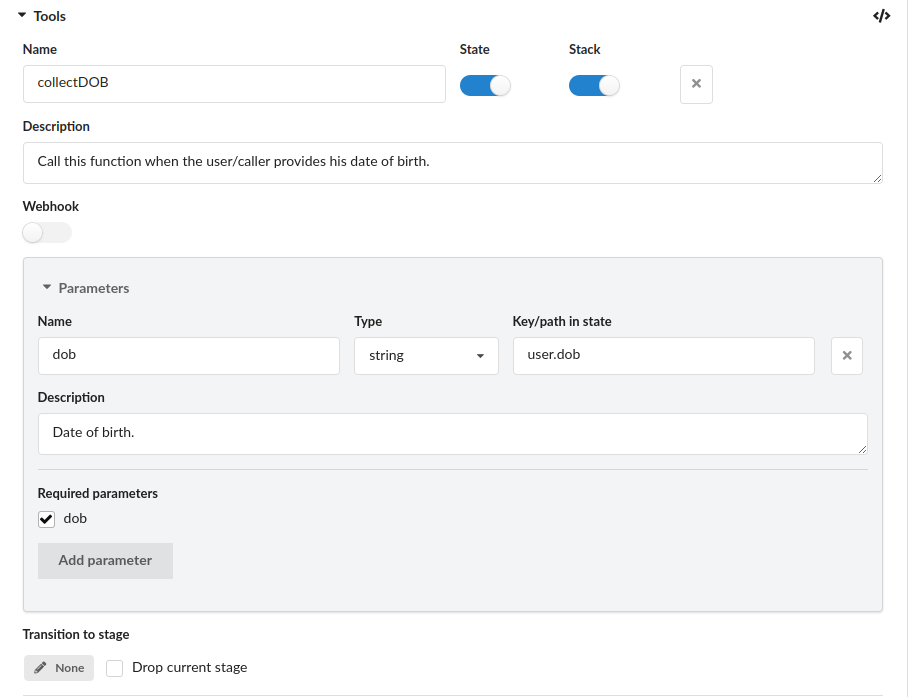
Finally, we need to provide the implementation of the collectDOB function that we defined in the tools section. In our example, we simply reply with "Thank you!" and hangup the call.
function collectDOB(params) {
return { text: "Thank you!", action: "hangup" };
}
Test Out The Bot Using The Playground
As we can see from the conversation below, the bot greeted the caller, and asked for his/her name and his/her birthday. Once the user/caller provided the required information, the bot responded with thank you repeating the provided name.
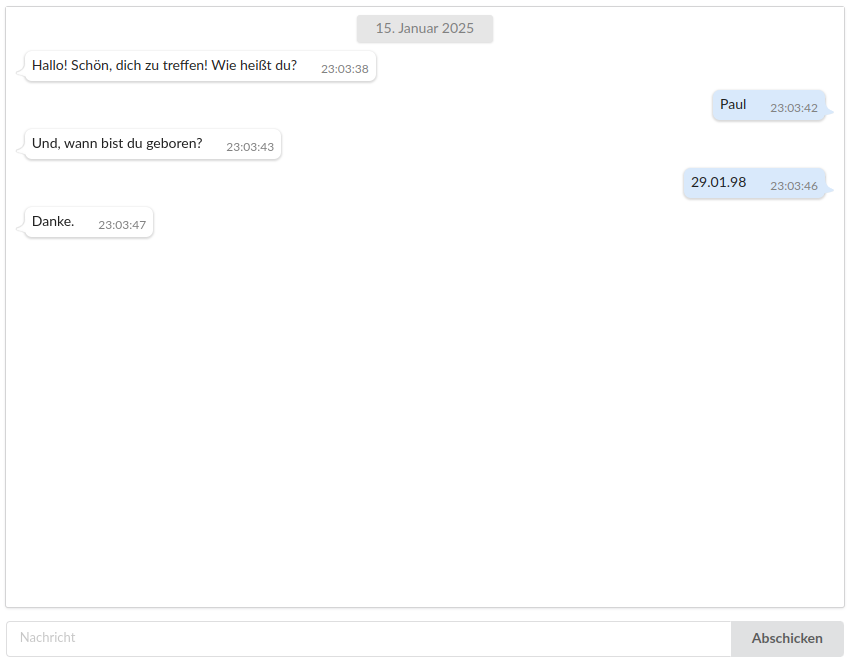